mirror of
https://github.com/hubHarmony/servii-backend.git
synced 2024-11-17 21:40:31 +00:00
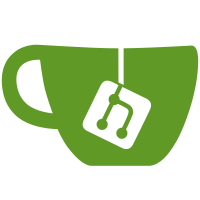
Now uses firebase complete tokens; it verifies the signature and integrity of the token, the origin of the project the token was issued for, the secret key, and finally the sub before verifying the account.
250 lines
11 KiB
HTML
250 lines
11 KiB
HTML
<!DOCTYPE html>
|
|
<html lang="en">
|
|
<head>
|
|
<meta charset="UTF-8">
|
|
<title>File Upload and API Interaction Form</title>
|
|
<style>
|
|
body {
|
|
font-family: Arial, sans-serif;
|
|
max-width: 600px;
|
|
margin: 0 auto;
|
|
padding: 20px;
|
|
background-color: #f0f0f0;
|
|
}
|
|
form {
|
|
display: flex;
|
|
flex-direction: column;
|
|
gap: 15px;
|
|
background-color: #ffffff;
|
|
padding: 20px;
|
|
border-radius: 5px;
|
|
box-shadow: 0 0 10px rgba(0,0,0,0.1);
|
|
}
|
|
input[type="file"], input[type="text"], input[type="number"] {
|
|
padding: 10px;
|
|
margin-bottom: 10px;
|
|
border: 1px solid #ccc;
|
|
border-radius: 4px;
|
|
}
|
|
button {
|
|
padding: 12px;
|
|
background-color: #007bff;
|
|
color: white;
|
|
border: none;
|
|
border-radius: 5px;
|
|
cursor: pointer;
|
|
transition: background-color 0.3s ease;
|
|
}
|
|
button:hover {
|
|
background-color: #0056b3;
|
|
}
|
|
.message {
|
|
margin-top: 10px;
|
|
padding: 12px;
|
|
border-radius: 4px;
|
|
}
|
|
.success {
|
|
background-color: #d4edda;
|
|
color: #155724;
|
|
border: 1px solid #c3e6cb;
|
|
}
|
|
.error {
|
|
background-color: #f8d7da;
|
|
color: #721c24;
|
|
border: 1px solid #f5c6cb;
|
|
}
|
|
</style>
|
|
</head>
|
|
<body>
|
|
|
|
<h2>File Upload Form</h2>
|
|
<form id="uploadForm">
|
|
<input type="file" id="files" accept=".zip,.jar,.txt" multiple required>
|
|
<button type="submit">Upload File</button>
|
|
</form>
|
|
|
|
<div id="message" class="message"></div>
|
|
|
|
<h2>API Interaction Form</h2>
|
|
<form id="genericForm">
|
|
Email: <input type="text" id="accountEmail"><br>
|
|
Port: <input type="number" id="accountPort"><br>
|
|
Name: <input type="text" id="serverName"><br>
|
|
Version: <input type="text" id="serverVersion"><br>
|
|
Framework: <input type="text" id="serverFramework"><br>
|
|
<button type="button" class="actionButton" data-action="AccountCreate">Create Account</button>
|
|
<button type="button" class="actionButton" data-action="AccountDelete">Delete Account</button>
|
|
<button type="button" class="actionButton" data-action="ServerCreate">Create Server</button>
|
|
<button type="button" class="actionButton" data-action="ServerDelete">Delete Server</button>
|
|
<button type="button" class="actionButton" data-action="ServerRun">Start Server</button>
|
|
<button type="button" class="actionButton" data-action="ServerStop">Stop Server</button>
|
|
<button type="button" class="actionButton" data-action="FetchServers">Fetch Servers</button>
|
|
<button type="button" class="actionButton" data-action="FetchLogs">Fetch Logs</button>
|
|
<button type="button" class="actionButton" data-action="FetchPlayersStatus">Fetch Players Status</button>
|
|
<button type="button" class="actionButton" data-action="FetchDirContent">Fetch Directory Content</button>
|
|
</form>
|
|
|
|
<h2>Update Property</h2>
|
|
<form id="updatePropertyForm">
|
|
Property: <input type="text" id="update_property"><br>
|
|
Value: <input type="text" id="update_value"><br>
|
|
<button type="button" class="actionButton" data-action="UpdateProperties">Update Property</button>
|
|
</form>
|
|
|
|
<h2>Send Command</h2>
|
|
<form id="sendCommandForm">
|
|
Command: <input type="text" id="command"><br>
|
|
<button type="button" class="actionButton" data-action="Command">Send command</button>
|
|
</form>
|
|
|
|
<h2>Set Subdomain</h2>
|
|
<form id="setSubdomainForm">
|
|
Subdomain: <input type="text" id="subdomain"><br>
|
|
<button type="button" class="actionButton" data-action="SetSubdomain">Set Subdomain</button>
|
|
</form>
|
|
|
|
<script>
|
|
document.addEventListener('DOMContentLoaded', () => {
|
|
const uploadForm = document.getElementById('uploadForm');
|
|
const messageDiv = document.getElementById('message');
|
|
const token = "eyJhbGciOiJSUzI1NiIsImtpZCI6ImUwM2E2ODg3YWU3ZjNkMTAyNzNjNjRiMDU3ZTY1MzE1MWUyOTBiNzIiLCJ0eXAiOiJKV1QifQ.eyJuYW1lIjoiSXR6IFNlbiIsInBpY3R1cmUiOiJodHRwczovL2xoMy5nb29nbGV1c2VyY29udGVudC5jb20vYS9BQ2c4b2NLOElVZHlzcW5kZkxxNFc5ZWlRNlpjTFpkbUVDX29UNXBVaURGQ2gzY2VDZTZXSGxvWD1zOTYtYyIsImlzcyI6Imh0dHBzOi8vc2VjdXJldG9rZW4uZ29vZ2xlLmNvbS9zZXJ2aS1lNjcwNSIsImF1ZCI6InNlcnZpLWU2NzA1IiwiYXV0aF90aW1lIjoxNzI2ODM5NTUwLCJ1c2VyX2lkIjoiTXBrYkRNT084UFFkZFFnQjVWZ0JRZFRNV0Y1MyIsInN1YiI6Ik1wa2JETU9POFBRZGRRZ0I1VmdCUWRUTVdGNTMiLCJpYXQiOjE3MjY4Mzk1NTAsImV4cCI6MTcyNjg0MzE1MCwiZW1haWwiOiJ0ZWNobm9wcm9kMjU0NTg1NjVAZ21haWwuY29tIiwiZW1haWxfdmVyaWZpZWQiOnRydWUsImZpcmViYXNlIjp7ImlkZW50aXRpZXMiOnsiZ29vZ2xlLmNvbSI6WyIxMTQ0Mzk0NjEyOTM5OTE1NzU5MTgiXSwiZW1haWwiOlsidGVjaG5vcHJvZDI1NDU4NTY1QGdtYWlsLmNvbSJdfSwic2lnbl9pbl9wcm92aWRlciI6Imdvb2dsZS5jb20ifX0.Aa3mdAmOzYET_QsGk2-QKxLGhxtXGfyAcTRnnM6cPGx0UJeSoQ-EhMIgK7HDiLVni_eMHbnwMSeEXDHEpsCWosm6e3e96zwMU3GXI1nowcnZ3CYTDH8jDCs2-6_ODomZtT2S1Lp3fD7IoSD4tDGFdo9kZNyuFGApTHhFHNAyHvfBqGL_c0c71Gfh-6ywl5C8nc07YPVbYGJu6GrS28L1vOjRSkl89Xm7o6atf38YWYWwg84QsrugRlF7Nz6yZJf7cjRY5x2guilqxrWVCWhlLiCMqFhe4oIW3BL7s3AfUC6U7DvlTyGwZJoN3fUr7V1Q5xloqSz7dcexRe1YkXXrCA";
|
|
|
|
|
|
// File Upload functionality
|
|
uploadForm.addEventListener('submit', async event => {
|
|
event.preventDefault();
|
|
const fileInput = document.getElementById('files');
|
|
const filesLength = fileInput.files.length;
|
|
|
|
if (filesLength === 0) {
|
|
showMessage('Please select at least one file.', 'error');
|
|
return;
|
|
}
|
|
|
|
const formData = new FormData();
|
|
formData.append('name', 'local');
|
|
formData.append('token', 'MpkbDMOO8PQddQgB5VgBQdTMWF53');
|
|
for (let i = 0; i < filesLength; i++) {
|
|
formData.append(`${i}`, fileInput.files[i]);
|
|
}
|
|
|
|
try {
|
|
const response = await fetch('http://localhost:3000/Upload', {
|
|
method: 'POST',
|
|
headers: {'SST': token},
|
|
body: formData
|
|
});
|
|
|
|
if (!response.ok) {
|
|
const errorMessage = await response.text();
|
|
const parser = new DOMParser();
|
|
const doc = parser.parseFromString(errorMessage, 'text/html');
|
|
const title = doc.querySelector('title')?.textContent || 'Unknown Error';
|
|
const description = doc.querySelector('p')?.textContent || 'Unable to determine error description.';
|
|
|
|
showMessage(`${title}: ${description}`, 'error');
|
|
} else {
|
|
const result = await response.json();
|
|
showMessage(`File uploaded successfully. Filename: ${result.filename}`, 'success');
|
|
}
|
|
} catch (error) {
|
|
console.error('Error:', error);
|
|
showMessage('An error occurred. Please try again.', 'error');
|
|
}
|
|
});
|
|
|
|
// API Interaction functionality
|
|
const buttons = document.querySelectorAll('.actionButton');
|
|
|
|
buttons.forEach(button => {
|
|
button.addEventListener('click', async event => {
|
|
event.preventDefault();
|
|
const action = button.dataset.action;
|
|
//const token = "dhmNGJYaVzNkKWgMAEOoAjaPWdc2";
|
|
const token = "eyJhbGciOiJSUzI1NiIsImtpZCI6ImUwM2E2ODg3YWU3ZjNkMTAyNzNjNjRiMDU3ZTY1MzE1MWUyOTBiNzIiLCJ0eXAiOiJKV1QifQ.eyJuYW1lIjoiSXR6IFNlbiIsInBpY3R1cmUiOiJodHRwczovL2xoMy5nb29nbGV1c2VyY29udGVudC5jb20vYS9BQ2c4b2NLOElVZHlzcW5kZkxxNFc5ZWlRNlpjTFpkbUVDX29UNXBVaURGQ2gzY2VDZTZXSGxvWD1zOTYtYyIsImlzcyI6Imh0dHBzOi8vc2VjdXJldG9rZW4uZ29vZ2xlLmNvbS9zZXJ2aS1lNjcwNSIsImF1ZCI6InNlcnZpLWU2NzA1IiwiYXV0aF90aW1lIjoxNzI2ODM5NTUwLCJ1c2VyX2lkIjoiTXBrYkRNT084UFFkZFFnQjVWZ0JRZFRNV0Y1MyIsInN1YiI6Ik1wa2JETU9POFBRZGRRZ0I1VmdCUWRUTVdGNTMiLCJpYXQiOjE3MjY4Mzk1NTAsImV4cCI6MTcyNjg0MzE1MCwiZW1haWwiOiJ0ZWNobm9wcm9kMjU0NTg1NjVAZ21haWwuY29tIiwiZW1haWxfdmVyaWZpZWQiOnRydWUsImZpcmViYXNlIjp7ImlkZW50aXRpZXMiOnsiZ29vZ2xlLmNvbSI6WyIxMTQ0Mzk0NjEyOTM5OTE1NzU5MTgiXSwiZW1haWwiOlsidGVjaG5vcHJvZDI1NDU4NTY1QGdtYWlsLmNvbSJdfSwic2lnbl9pbl9wcm92aWRlciI6Imdvb2dsZS5jb20ifX0.Aa3mdAmOzYET_QsGk2-QKxLGhxtXGfyAcTRnnM6cPGx0UJeSoQ-EhMIgK7HDiLVni_eMHbnwMSeEXDHEpsCWosm6e3e96zwMU3GXI1nowcnZ3CYTDH8jDCs2-6_ODomZtT2S1Lp3fD7IoSD4tDGFdo9kZNyuFGApTHhFHNAyHvfBqGL_c0c71Gfh-6ywl5C8nc07YPVbYGJu6GrS28L1vOjRSkl89Xm7o6atf38YWYWwg84QsrugRlF7Nz6yZJf7cjRY5x2guilqxrWVCWhlLiCMqFhe4oIW3BL7s3AfUC6U7DvlTyGwZJoN3fUr7V1Q5xloqSz7dcexRe1YkXXrCA";
|
|
const framework = document.getElementById('serverFramework').value;
|
|
const subdomain = document.getElementById('subdomain').value;
|
|
const email = document.getElementById('accountEmail').value;
|
|
const port = document.getElementById('accountPort').value;
|
|
const name = document.getElementById('serverName').value;
|
|
const version = document.getElementById('serverVersion').value;
|
|
const prop = document.getElementById('update_property').value;
|
|
const value = document.getElementById('update_value').value;
|
|
const command = document.getElementById('command').value;
|
|
const props = [[prop, value], ["max-players", "666"]];
|
|
let data = {};
|
|
|
|
switch(action) {
|
|
case 'FetchServers':
|
|
data = {};
|
|
break;
|
|
case 'FetchLogs':
|
|
data = {name};
|
|
break;
|
|
case 'FetchPlayersStatus':
|
|
data = { name};
|
|
break;
|
|
case 'FetchDirContent':
|
|
data = { name};
|
|
break;
|
|
case 'AccountCreate':
|
|
data = {email, port, };
|
|
break;
|
|
case 'AccountDelete':
|
|
data = {subdomain, port, };
|
|
break;
|
|
case 'ServerCreate':
|
|
data = {port, name, version, framework};
|
|
break;
|
|
case 'ServerDelete':
|
|
data = {port, name, };
|
|
break;
|
|
case 'ServerRun':
|
|
data = {port, name, };
|
|
break;
|
|
case 'ServerStop':
|
|
data = {port, name, };
|
|
break;
|
|
case 'UpdateProperties':
|
|
data = {port, name, props, value, };
|
|
break;
|
|
case 'Command':
|
|
data = {port, name, command, };
|
|
break;
|
|
case 'SetSubdomain':
|
|
data = {subdomain};
|
|
break;
|
|
}
|
|
|
|
sendRequest(action, data, token)
|
|
.then(response => response.text())
|
|
.then(data => alert(`Response: ${data}`))
|
|
.catch(error => console.error('Error:', error));
|
|
});
|
|
});
|
|
|
|
function sendRequest(endpoint, payload, token) {
|
|
return fetch(`http://localhost:3000/${endpoint}`, {
|
|
method: 'POST',
|
|
headers: {
|
|
'Content-Type': 'application/json',
|
|
'SST': token,
|
|
},
|
|
body: JSON.stringify(payload)
|
|
});
|
|
}
|
|
|
|
function showMessage(message, type) {
|
|
messageDiv.textContent = message;
|
|
messageDiv.className = `message ${type}`;
|
|
setTimeout(() => {
|
|
messageDiv.textContent = '';
|
|
messageDiv.className ='message';
|
|
}, 5000);
|
|
}
|
|
});
|
|
</script>
|
|
|
|
</body>
|
|
</html>
|