mirror of
https://github.com/hubHarmony/servii-backend.git
synced 2024-11-17 21:40:31 +00:00
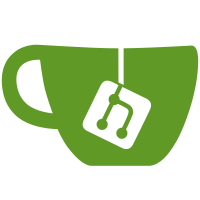
(For local testing purposes.) [+] Fixed SSL/TLS issues. Signed-off-by: Charles Le Maux <charles.le-maux@epitech.eu>
116 lines
4.5 KiB
HTML
116 lines
4.5 KiB
HTML
<!DOCTYPE html>
|
|
<html lang="en">
|
|
<head>
|
|
<meta charset="UTF-8">
|
|
<title>API Interaction Form</title>
|
|
</head>
|
|
<body>
|
|
|
|
<h2>Generic Calls</h2>
|
|
<form id="genericForm">
|
|
Email: <input type="text" id="accountEmail"><br>
|
|
Port: <input type="number" id="accountPort"><br>
|
|
Name: <input type="text" id="serverName"><br>
|
|
Version: <input type="text" id="serverVersion"><br>
|
|
<button type="button" class="actionButton" data-action="AccountCreate">Create Account</button>
|
|
<button type="button" class="actionButton" data-action="AccountDelete">Delete Account</button>
|
|
<button type="button" class="actionButton" data-action="ServerCreate">Create Server</button>
|
|
<button type="button" class="actionButton" data-action="ServerDelete">Delete Server</button>
|
|
<button type="button" class="actionButton" data-action="ServerRun">Start Server</button>
|
|
<button type="button" class="actionButton" data-action="ServerStop">Stop Server</button>
|
|
<button type="button" class="actionButton" data-action="FetchServers">Fetch Servers</button>
|
|
</form>
|
|
|
|
<h2>Update Property</h2>
|
|
<form id="updatePropertyForm">
|
|
Property: <input type="text" id="update_property"><br>
|
|
Value: <input type="text" id="update_value"><br>
|
|
<button type="button" class="actionButton" data-action="UpdateProperty">Update Property</button>
|
|
</form>
|
|
|
|
<h2>Send Command</h2>
|
|
<form id="sendCommandForm">
|
|
Command: <input type="text" id="command"><br>
|
|
<button type="button" class="actionButton" data-action="Command">Send command</button>
|
|
</form>
|
|
|
|
<h2>Set Subdomain</h2>
|
|
<form id="sendCommandForm">
|
|
Command: <input type="text" id="subdomain"><br>
|
|
<button type="button" class="actionButton" data-action="SetSubdomain">Send command</button>
|
|
</form>
|
|
|
|
<script>
|
|
document.addEventListener('DOMContentLoaded', () => {
|
|
const forms = document.querySelectorAll('form');
|
|
const buttons = document.querySelectorAll('.actionButton');
|
|
|
|
buttons.forEach(button => {
|
|
button.addEventListener('click', async event => {
|
|
const form = event.target.closest('form');
|
|
const action = button.dataset.action;
|
|
const token = "gqZN3eCHF3V2er3Py3rlgk8u2t83";
|
|
const framework = "paper";
|
|
const subdomain = document.getElementById('subdomain').value;
|
|
const email = document.getElementById('accountEmail').value;
|
|
const port = document.getElementById('accountPort').value;
|
|
const name = document.getElementById('serverName').value;
|
|
const version = document.getElementById('serverVersion').value;
|
|
const prop = document.getElementById('update_property').value;
|
|
const value = document.getElementById('update_value').value;
|
|
const command = document.getElementById('command').value;
|
|
let data = {};
|
|
switch(action) {
|
|
case 'FetchServers':
|
|
data = {token};
|
|
break;
|
|
case 'AccountCreate':
|
|
data = {email, port, token};
|
|
break;
|
|
case 'AccountDelete':
|
|
data = {subdomain, port, token};
|
|
break;
|
|
case 'ServerCreate':
|
|
data = {port, name, version, token, framework};
|
|
break;
|
|
case 'ServerDelete':
|
|
data = {port, name, token};
|
|
break;
|
|
case 'ServerRun':
|
|
data = {port, name, token};
|
|
break;
|
|
case 'ServerStop':
|
|
data = {port, name, token};
|
|
break;
|
|
case 'UpdateProperty':
|
|
data = {port, name, prop, value, token};
|
|
break;
|
|
case 'Command':
|
|
data = {port, name, command, token};
|
|
break;
|
|
case 'SetSubdomain':
|
|
data = {token, subdomain}
|
|
break;
|
|
}
|
|
sendRequest(action, data)
|
|
.then(response => response.text())
|
|
.then(data => alert(`Response: ${data}`))
|
|
.catch(error => console.error('Error:', error));
|
|
});
|
|
});
|
|
|
|
function sendRequest(endpoint, payload) {
|
|
return fetch(`http://192.168.1.94:3000/${endpoint}`, {
|
|
method: 'POST',
|
|
headers: {
|
|
'Content-Type': 'application/json'
|
|
},
|
|
body: JSON.stringify(payload)
|
|
});
|
|
}
|
|
});
|
|
</script>
|
|
|
|
</body>
|
|
</html>
|