2018-08-27 14:16:18 +00:00
# Migrating a project from UNet (HLAPI) #
This guide gives you a step by step instruction for migrating your project from HLAP to Mirror.
Mirror is a fork of HLAPI. As such the migration is straight forward for most projects.
## 1) BACKUP ##
2018-08-28 08:55:45 +00:00
You have been warned.
2018-08-27 14:16:18 +00:00
## 2) Install Mirror ##
Get Mirror from the asset store and import it in your project (link to be provided)
## 3) Replace namespace ##
Replace `Unity.Networking` for `Mirror` everywhere in your project. So for example, if you have this:
```
using Unity.Networking;
public class Player : NetworkBehaviour {
...
}
```
you would replace it with:
```
using Mirror;
public class Player : NetworkBehaviour {
...
}
```
At this point, you might get some compilation errors. Don't panic, these are easy to fix. Keep going
## 4) Remove all channels ##
2018-08-28 08:55:45 +00:00
As of this writing, all messages are reliable, ordered, fragmented. There is no need for channels at all.
To avoid making misleading code, we have removed channels.
2018-08-27 14:16:18 +00:00
For example, if you have this code:
```
2018-08-29 13:00:04 +00:00
[Command(channel= Channels.DefaultReliable)]
2018-08-27 14:16:18 +00:00
public void CmdMove(int x, int y)
{
...
}
```
replace it with:
```
[Command]
public void CmdMove(int x, int y)
{
...
}
```
The same applies for `[ClientRPC]` , `[NetworkSettings]` , `[SyncEvent]` , `[SyncVar]` , `[TargetRPC]`
2018-08-28 08:55:45 +00:00
## 5) Rename SyncListStruct to SyncListSTRUCT ##
2018-08-29 13:04:58 +00:00
There is a bug in the original UNET Weaver that makes it mess with our `Mirror.SyncListStruct` without checking the namespace.
Until Unity officially removes UNET in 2019.1, we will have to use the name `SyncListSTRUCT` instead.
2018-08-28 08:55:45 +00:00
So for example, if you have definitions like:
```
public class SyncListQuest : SyncListStruct< Quest > {}
```
replace them with:
```
public class SyncListQuest : SyncListSTRUCT< Quest > {}
```
## 6. Replace Components ##
2018-08-27 14:33:39 +00:00
Every networked prefab and scene object needs to be adjusted. They will be using `NetworkIdentity` from Unet, and you need to replace that componenent with `NetworkIdentity` from Mirror. You may be using other network components, such as `NetworkAnimator` or `NetworkTransform` . All components from Unet should be replaced with their corresponding component from Mirror.
2018-08-28 21:16:41 +00:00
Note that if you remove and add a NetworkIdentity, you will need to reassign it in any component that was referencing it.
2018-08-29 13:04:58 +00:00
## 7. Update your firewall and router ##
2018-08-27 14:16:18 +00:00
LLAPI uses UDP. Mirror uses TCP by default. This means you may need to change your router
2018-08-29 13:04:58 +00:00
port forwarding and firewall rules in your machine to expose the TCP port instead of UDP.
This highly depends on your router and operating system.
2018-08-28 09:01:17 +00:00
2018-08-28 21:16:41 +00:00
# Video version #
See for yourself how uMMORPG was migrated to Mirror
[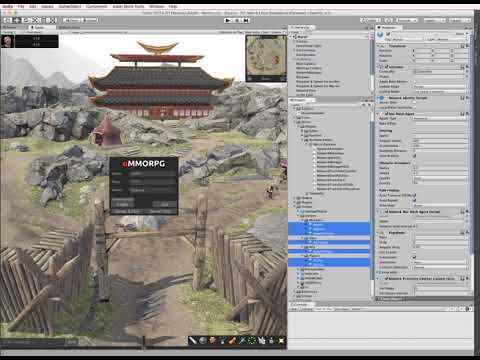](http://www.youtube.com/watch?v=LF9rTSS3rlI)
2018-08-28 09:01:17 +00:00
## Possible Error Messages
2018-08-28 21:16:41 +00:00
* TypeLoadException: A type load exception has occurred. - happens if you still have SyncListStruct instead of SyncListSTRUCT in your project.
2018-08-29 13:04:58 +00:00
* NullPointerException: The most likely cause is that you replaced NetworkIdentities or other components but you had them assigned somewhere. Reassign those references.
2018-08-29 15:33:40 +00:00
2018-08-29 15:38:38 +00:00
* `error CS0246: The type or namespace name 'UnityWebRequest' could not be found. Are you missing 'UnityEngine.Networking' using directive?`
2018-08-29 15:33:40 +00:00
Add this to the top of your script:
```
using UnityWebRequest = UnityEngine.Networking.UnityWebRequest ;
```
`UnityWebRequest` is not part of UNet or Mirror, but it is in the same namespace as UNet, so the namespace change might cause your script not to find UnityWebRequest.