mirror of
https://github.com/MirrorNetworking/Mirror.git
synced 2024-11-18 11:00:32 +00:00
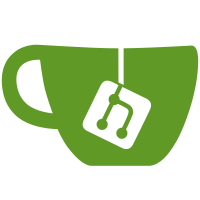
* Documentation Outline * Spacing adjustments * Captured old wiki content * yml fix * Docs work * resize images * Replaced images * Removed md from links * Renamed Misty to Fizzy * Captured Unity docs * links cleanup * clear links * Cleanup and moved NetworkBehavior to Classes. * added slashes to yml paths * reverted slashes * Fixes bad link * Update Ignorance.md This should be enough documentation for now, yeah? * Localized images * Update Ignorance.md formatting updates * Lots of Cleanup * fix link * Formatting * fix code blocks * Lots of content and cleanup * fixed yml * Added blank line * Added spaces in titles * tightened bullets * Fixed bullet spacing * Fixed more bullets * unbolded content * Cleanup and removal of empty pages Updated README with links to docs pages * Restored prior version * Contributing * Improvements to content * lower case fix * fix link * renamed Contributions * fixed link * home page content * Fixed Encoding * Moved Why TCP * Replaced Unity with Mirror * Telepathy Description * changed to h2 * Moved Sample down * Removed dead links * Copied Contributions Added Test Fixed h3's * Fixed headings * added to Test * Fixed image alts and links * fixed last alt
78 lines
2.4 KiB
Markdown
78 lines
2.4 KiB
Markdown
# Messages Overview
|
|
|
|
General description of Messages
|
|
|
|
- **AddPlayerMessage**
|
|
This is passed to handler functions registered for the AddPlayer built-in message.
|
|
- **EmptyMessage**
|
|
A utility class to send a network message with no contents.
|
|
|
|
```cs
|
|
using UnityEngine;
|
|
using Mirror;
|
|
|
|
public class Test
|
|
{
|
|
void SendNotification()
|
|
{
|
|
var msg = new EmptyMessage();
|
|
NetworkServer.SendToAll(667, msg);
|
|
}
|
|
}
|
|
```
|
|
|
|
- **ErrorMessage**
|
|
This is passed to handler functions registered for the SYSTEM_ERROR built-in message.
|
|
- **IntegerMessage**
|
|
A utility class to send simple network messages that only contain an integer.
|
|
|
|
```cs
|
|
using UnityEngine;
|
|
using Mirror;
|
|
|
|
public class Test
|
|
{
|
|
void SendValue(int value)
|
|
{
|
|
var msg = new IntegerMessage(value);
|
|
NetworkServer.SendToAll(MsgType.Scene, msg);
|
|
}
|
|
}
|
|
```
|
|
|
|
- **NotReadyMessage**
|
|
This is passed to handler functions registered for the SYSTEM_NOT_READY built-in message.
|
|
- **PeerAuthorityMessage**
|
|
Information about a change in authority of a non-player in the same network game.
|
|
This information is cached by clients and used during host-migration.
|
|
- **PeerInfoMessage**
|
|
Information about another participant in the same network game.
|
|
This information is cached by clients and used during host-migration.
|
|
- **PeerInfoPlayer**
|
|
A structure used to identify player object on other peers for host migration.
|
|
- **PeerListMessage**
|
|
Internal UNET message for sending information about network peers to clients.
|
|
- **ReadyMessage**
|
|
This is passed to handler functions registered for the SYSTEM_READY built-in message.
|
|
- **ReconnectMessage**
|
|
This network message is used when a client reconnect to the new host of a game.
|
|
- **RemovePlayerMessage**
|
|
This is passed to handler funtions registered for the SYSTEM_REMOVE_PLAYER built-in message.
|
|
- **StringMessage**
|
|
This is a utility class for simple network messages that contain only a string.
|
|
This example sends a message with the name of the Scene.
|
|
|
|
```cs
|
|
using UnityEngine;
|
|
using Mirror;
|
|
|
|
public class Test
|
|
{
|
|
void SendSceneName(string sceneName)
|
|
{
|
|
var msg = new StringMessage(sceneName);
|
|
NetworkServer.SendToAll(MsgType.Scene, msg);
|
|
}
|
|
}
|
|
```
|