mirror of
https://github.com/MirrorNetworking/Mirror.git
synced 2024-11-18 11:00:32 +00:00
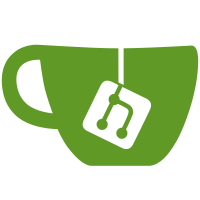
* Moved doc files to docfx folder * load csproj * doc generation * Run docfx * Add docfx * Deploy docs to mirror-networking.com * use deploy phase * deploy whole generated site * Fixed the semantic release command * Is last \ required? * show debug log * using lftp for site deploy * Testing lftp * Show current folder * try -e command option * Show me the files * use plain ftp * use choco install instead of cinst * fix ssl certificate validation * fix username * Upload site to xmldocs folder * no need to archive docs * No need for debug output * Fix file permissions * show me .htaccess * Show me contents * Wipe out folder to fix permissions * Set file permissions * Fix file permissions * complete toc list * Migrated intro page * Remove old docs * Update link to docs * Add link to github * Only update docs for stuff in master * This is a powershell command * Update doc/articles/Concepts/Communications/RemoteActions.md * Update doc/articles/Concepts/VisibilityCustom.md * Update doc/articles/Concepts/Authority.md * Update doc/articles/Concepts/GameObjects/SpawnObjectCustom.md * Update doc/articles/Concepts/Authority.md * Update doc/articles/Classes/SyncVars.md * No need to run semver twice
49 lines
1.2 KiB
Markdown
49 lines
1.2 KiB
Markdown
# Clock Synchronization
|
|
|
|
For many algorithms you need the clock to be synchronized between the client and
|
|
the server. Mirror does that automatically for you.
|
|
|
|
To get the current time use this code:
|
|
|
|
```cs
|
|
double now = NetworkTime.time;
|
|
```
|
|
|
|
It will return the same value in the client and the servers. It starts at 0 when
|
|
the server starts. Note the time is a double and should never be casted to a
|
|
float. Casting this down to a float means the clock will lose precision after
|
|
some time:
|
|
|
|
- after 1 day, accuracy goes down to 8 ms
|
|
- after 10 days, accuracy is 62 ms
|
|
- after 30 days , accuracy is 250 ms
|
|
- after 60 days, accuracy is 500 ms
|
|
|
|
Mirror will also calculate the RTT time as seen by the application:
|
|
|
|
```cs
|
|
double rtt = NetworkTime.rtt;
|
|
```
|
|
|
|
You can measure accuracy.
|
|
|
|
```cs
|
|
double time_standard_deviation = NetworkTime.timeSd;
|
|
```
|
|
|
|
for example, if this returns 0.2, it means the time measurements swing up and
|
|
down roughly 0.2 s
|
|
|
|
Network hickups are compensated against by smoothing out the values using EMA.
|
|
You can configure how often you want the the ping to be sent:
|
|
|
|
```cs
|
|
NetworkTime.PingFrequency = 2.0f;
|
|
```
|
|
|
|
You can also configure how many ping results are used in the calculation:
|
|
|
|
```cs
|
|
NetworkTime.PingWindowSize = 10;
|
|
```
|