mirror of
https://github.com/MirrorNetworking/Mirror.git
synced 2024-11-18 19:10:32 +00:00
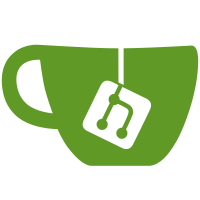
* Added basic SyncDictionary support, no support for structs yet * Fixed TryGetValue usage * Removed extraneous hardcoded SyncDictionary type * Added a couple basic tests, more coming * Added 4 more tests * Added two tests and SyncDictionary now bubbles item to Callback on Remove (both Remove cases) * Added the remainder of tests * Added basic documentation about SyncDictionaries on StateSync.md page * Simplify test syntax Co-Authored-By: Katori <znorth@gmail.com> * Simplify test syntax Co-Authored-By: Katori <znorth@gmail.com> * Simplify test syntax Co-Authored-By: Katori <znorth@gmail.com> * Simplify test syntax Co-Authored-By: Katori <znorth@gmail.com> * Remove null-check when setting value directly (and updated expected test behaviour) * fix: Provide default implementation for SyncDictionary serializers * feat: Add Weaver support for syncdictionary * Fix minor issue with Set code and made test use Weaved serialization instead of manual * Added a new test for bare set (non-overwrite) * Added another test for BareSetNull and cleaned up some tests * Updated SyncDictionary documentation on StateSync.md * Update docs with SyncDictionary info * Update SyncDictionary docs wording * docs: document the types and better example * Add two SyncDictionary constructors * Removed unnecessary initialization * Style fixes * - Merged many operation cases - Fixed Contains method - Added new test to test contains (and flag its earlier improper usage) - Use PackedUInt32 instead of int for Changes and Counts * - Simplify "default" syntax - Use Rodol's remove method (faster) - Don't use var * Removed unnecessary newline, renamed <B, T> to <K, V> per vis2k, corrected wording of InvalidOperationException on ReadOnly AddOp * Code simplification, style fixes, docs example style fixes, newly improved implementation for CopyTo that fails gracefully
2.0 KiB
2.0 KiB
SyncDictionary
A SyncDictionary
is an associative array containing an unordered list of key, value pairs. Keys and values can be of the following types:
- Basic type (byte, int, float, string, UInt64, etc)
- Built-in Unity math type (Vector3, Quaternion, etc)
- NetworkIdentity
- GameObject with a NetworkIdentity component attached.
- Struct with any of the above
SyncDictionaries work much like SyncLists: when you make a change on the server the change is propagated to all clients and the Callback is called.
To use it, create a class that derives from SyncDictionary
for your specific type. This is necesary because the Weaver will add methods to that class. Then add a field to your NetworkBehaviour class.
Simple Example
using UnityEngine;
using Mirror;
public class ExamplePlayer : NetworkBehaviour
{
public class SyncDictionaryStringItem : SyncDictionary<string, Item> {}
public struct Item
{
public string name;
public int hitPoints;
public int durability;
}
public SyncDictionaryStringItem Equipment = new SyncDictionaryStringItem();
public void OnStartServer()
{
Equipment.Add("head", new Item { name = "Helmet", hitPoints = 10, durability = 20 });
Equipment.Add("body", new Item { name = "Epic Armor", hitPoints = 50, durability = 50 });
Equipment.Add("feet", new Item { name = "Sneakers", hitPoints = 3, durability = 40 });
Equipment.Add("hands", new Item { name = "Sword", hitPoints = 30, durability = 15 });
}
private void OnStartClient()
{
// Equipment is already populated with anything the server set up
// but we can subscribe to the callback in case it is updated later on
Equipment.Callback += OnEquipmentChange;
}
private void OnEquipmentChange(SyncDictionaryStringItem.Operation op, string key, Item item)
{
// equipment changed, perhaps update the gameobject
Debug.Log(op + " - " + key);
}
}