mirror of
https://github.com/MirrorNetworking/Mirror.git
synced 2024-11-18 11:00:32 +00:00
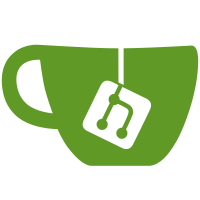
* Documentation Outline * Spacing adjustments * Captured old wiki content * yml fix * Docs work * resize images * Replaced images * Removed md from links * Renamed Misty to Fizzy * Captured Unity docs * links cleanup * clear links * Cleanup and moved NetworkBehavior to Classes. * added slashes to yml paths * reverted slashes * Fixes bad link * Update Ignorance.md This should be enough documentation for now, yeah? * Localized images * Update Ignorance.md formatting updates * Lots of Cleanup * fix link * Formatting * fix code blocks * Lots of content and cleanup * fixed yml * Added blank line * Added spaces in titles * tightened bullets * Fixed bullet spacing * Fixed more bullets * unbolded content * Cleanup and removal of empty pages Updated README with links to docs pages * Restored prior version * Contributing * Improvements to content * lower case fix * fix link * renamed Contributions * fixed link * home page content * Fixed Encoding * Moved Why TCP * Replaced Unity with Mirror * Telepathy Description * changed to h2 * Moved Sample down * Removed dead links * Copied Contributions Added Test Fixed h3's * Fixed headings * added to Test * Fixed image alts and links * fixed last alt
49 lines
1.2 KiB
Markdown
49 lines
1.2 KiB
Markdown
# Clock Synchronization
|
|
|
|
For many algorithms you need the clock to be synchronized between the client and
|
|
the server. Mirror does that automatically for you.
|
|
|
|
To get the current time use this code:
|
|
|
|
```cs
|
|
double now = NetworkTime.time;
|
|
```
|
|
|
|
It will return the same value in the client and the servers. It starts at 0 when
|
|
the server starts. Note the time is a double and should never be casted to a
|
|
float. Casting this down to a float means the clock will lose precision after
|
|
some time:
|
|
|
|
- after 1 day, accuracy goes down to 8 ms
|
|
- after 10 days, accuracy is 62 ms
|
|
- after 30 days , accuracy is 250 ms
|
|
- after 60 days, accuracy is 500 ms
|
|
|
|
Mirror will also calculate the RTT time as seen by the application:
|
|
|
|
```cs
|
|
double rtt = NetworkTime.rtt;
|
|
```
|
|
|
|
You can measure accuracy.
|
|
|
|
```cs
|
|
double time_standard_deviation = NetworkTime.timeSd;
|
|
```
|
|
|
|
for example, if this returns 0.2, it means the time measurements swing up and
|
|
down roughly 0.2 s
|
|
|
|
Network hickups are compensated against by smoothing out the values using EMA.
|
|
You can configure how often you want the the ping to be sent:
|
|
|
|
```cs
|
|
NetworkTime.PingFrequency = 2.0f;
|
|
```
|
|
|
|
You can also configure how many ping results are used in the calculation:
|
|
|
|
```cs
|
|
NetworkTime.PingWindowSize = 10;
|
|
```
|