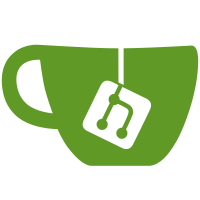
* Moved doc files to docfx folder * load csproj * doc generation * Run docfx * Add docfx * Deploy docs to mirror-networking.com * use deploy phase * deploy whole generated site * Fixed the semantic release command * Is last \ required? * show debug log * using lftp for site deploy * Testing lftp * Show current folder * try -e command option * Show me the files * use plain ftp * use choco install instead of cinst * fix ssl certificate validation * fix username * Upload site to xmldocs folder * no need to archive docs * No need for debug output * Fix file permissions * show me .htaccess * Show me contents * Wipe out folder to fix permissions * Set file permissions * Fix file permissions * complete toc list * Migrated intro page * Remove old docs * Update link to docs * Add link to github * Only update docs for stuff in master * This is a powershell command * Update doc/articles/Concepts/Communications/RemoteActions.md * Update doc/articles/Concepts/VisibilityCustom.md * Update doc/articles/Concepts/Authority.md * Update doc/articles/Concepts/GameObjects/SpawnObjectCustom.md * Update doc/articles/Concepts/Authority.md * Update doc/articles/Classes/SyncVars.md * No need to run semver twice
2.6 KiB
SyncSortedSet
SyncSortedSet
are sets similar to C# SortedSet<T> that synchronize their contents from the server to the clients.
Unlike SyncHashSets, all elements in a SyncSortedSet are sorted when they are inserted. Please note this has some performance implications.
A SyncSortedSet can contain items of the following types:
-
Basic type (byte, int, float, string, UInt64, etc)
-
Built-in Unity math type (Vector3, Quaternion, etc)
-
NetworkIdentity
-
Game object with a NetworkIdentity component attached.
-
Structure with any of the above
Usage
Create a class that derives from SyncSortedSet for your specific type. This is necessary because Mirror will add methods to that class with the weaver. Then add a SyncSortedSet field to your NetworkBehaviour class. For example:
class Player : NetworkBehaviour {
class SyncSkillSet : SyncSortedSet<string> {}
readonly SyncSkillSet skills = new SyncSkillSet();
int skillPoints = 10;
[Command]
public void CmdLearnSkill(string skillName)
{
if (skillPoints > 1)
{
skillPoints--;
skills.Add(skillName);
}
}
}
You can also detect when a SyncSortedSet changes. This is useful for refreshing your character in the client or determining when you need to update your database. Subscribe to the Callback event typically during Start
, OnClientStart
or OnServerStart
for that.
Note that by the time you subscribe, the set will already be initialized, so you will not get a call for the initial data, only updates.
Note SyncSets must be initialized in the constructor, not in Startxxx(). You can make them readonly to ensure correct usage.
class Player : NetworkBehaviour
{
class SyncSetBuffs : SyncSortedSet<string> {};
readonly SyncSetBuffs buffs = new SyncSetBuffs();
// this will add the delegate on the client.
// Use OnStartServer instead if you want it on the server
public override void OnStartClient()
{
buffs.Callback += OnBuffsChanged;
}
void OnBuffsChanged(SyncSetBuffs.Operation op, string buff)
{
switch (op)
{
case SyncSetBuffs.Operation.OP_ADD:
// we added a buff, draw an icon on the character
break;
case SyncSetBuffs.Operation.OP_CLEAR:
// clear all buffs from the character
break;
case SyncSetBuffs.Operation.OP_REMOVE:
// We removed a buff from the character
break;
}
}
}